What are the processes to build a React Native app?
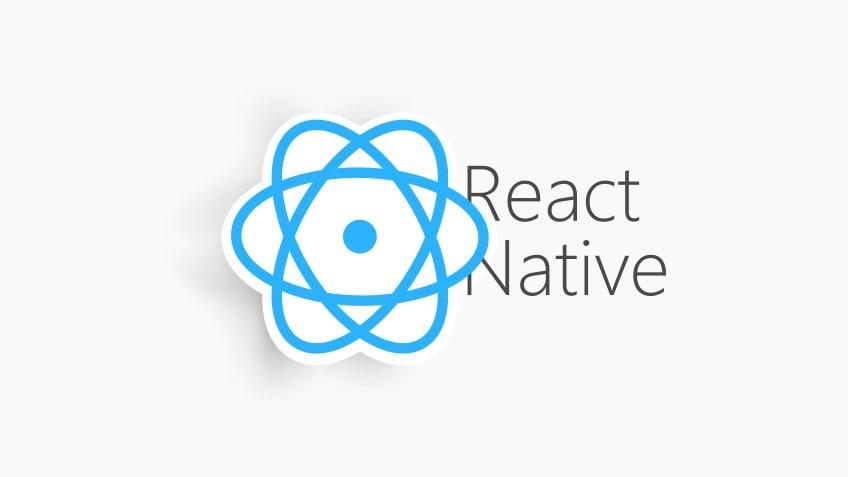
Hello, readership! Today, I want to talk a little about what is React Native, why it is important and popular now, and together with you I will try to write a small project to demonstrate the basics of the processes to build a React Native app.
What is React Native?
First of all, you need to figure out what React is. React is a tool for building user interfaces. Its main purpose is to ensure that what can be seen on web pages is displayed on the screen. React makes it easy to create interfaces by splitting each page into small chunks and components. It is very convenient for creating web applications and does not require a large threshold of entry. So the guys at Facebook thought it would be cool if React could be used to create cross-platform mobile apps, and in 2015 React Native was released to the public and appeared on GitHub, where everyone could contribute. React is popular for several reasons. It is compact and powerful, especially when dealing with rapidly changing data. Through its component structure, React encourages you to write modular and reusable code.
The world of cross-platform mobile development already had its own solutions, for example, Apache Cordova, a technology that allowed using HTML + CSS + JavaScript + native functionality of the platform on which the application was launched to run it. However, the technology has a big problem – performance. There are also others at the current moment, such as Xamarin, Flutter, QT and so on.
In React Native, code is written in JavaScript and executed using JavaScriptCore, the engine that uses Safari. You can also use native platform modules, such as a camera or Bluetooth. For this, code is written in such a way that implements the functionality in a language that is intended for development for a specific platform (Java/Swift /Objective C) and communicates with the JavaScript environment via a bridge.
Compared to typical iOS and Android development, React Native offers many other possibilities. Since your application is mostly JavaScript, there are many benefits to be gained. For example, in order to see the changes added to the code, you can immediately “update” the application, without waiting for the completion.
Most of the standard UI elements have components in RN, for example View = UIView on iOS, so it should be easy to implement interface sophistication if you have knowledge of React.
Why did React Native become so popular?
JavaScript is used to write code, in particular React. It is also possible to quickly write an application for both platforms. Less costs – more profitable for business. It has a large library of native and non-native components.
Besides, you can use the browser for debugging, there is also a hot-reload for quickly viewing changes. There is no need to rebuild the application when making changes to the code.
The amazing thing is that you can just write your own library for RN using the native functionality of the system.
Another reason why RN has gained popularity in recent years is that it is used by such giants as Facebook, Instagram, Skype, Tesla, Baidu, Bloomberg, etc.
The process to build a React Native application
Let’s try to write our example application and understand what is needed for this. Let it be, for example, an application for displaying and searching for different users.
We need the npx cli utility that comes with Node.js, Android Studio – if we want to test the application on Android, or Xcode – if on the iPhone. You can read more about how to install these tools on their official webpages.
Let’s start by creating a project with the npx react-native init RandomGuysApp command. You can also add TypeScript to an RN application, but we won’t do that here.
After some time, we created a folder structure.
In the console, write yarn add @ react-navigation / native @ react-navigation / stack react-native-reanimated react-native-gesture-handler react-native-screens react-native-safe-area-context @ react-native-community / masked-view.
This will install the navigation library so that you can navigate between application screens and dependencies for it. I decided not to include Redux, or another library for managing state, as I think that in our example it will be a little overkill. After installation, if you are going to run the application on iOS, you need to go to the ios folder and write pod install.
Index.js says:
AppRegistry.registerComponent (appName, () => App);
This shows that the App component is the entry point to our application. I prefer to keep the code in a separate folder, so I will move App.js to a new folder – src, changing the path to it in index.js to:
import {App} from './src/App';
Let’s start by creating a router. In the browser, when the user clicks on the link, the URL is added to the navigation history stack, and when the user clicks the back button, the browser removes the last visited address from the stack. In RN, there is no such concept by default, and that’s what React Navigation is for. An application can have multiple stacks. For example, each tab inside the application can have its own browsing history, but it can also have one.
Create a navigation folder, and in it create RootNavigator.js.
Let’s move on to creating the first screen. Add the screens folder, in which we create the PersonListScreen file and fill it with the following code:
import React, {Component} from 'react'; import {StyleSheet, TouchableOpacity, Image, Text, View} from 'react-native'; export class PersonListItem extends Component { render = () => { const {onPress, person} = this.props; return ( <TouchableOpacity style={styles.container} onPress = {onPress}> <Image source = {{uri: person.picture.medium}} resizeMode = {'contain'} style={styles.avatar} /> <View style={styles.col}> <Text style={styles.name}> {person.name.first} {person.name.last} </Text> <Text style={styles.email}> {person.email} </Text> </View> </TouchableOpacity> ); }; } const styles = StyleSheet.create ({ container: { flexDirection: 'row', alignItems: 'center', padding: 12, borderBottomColor: '# b0b0b0', borderBottomWidth: 0.4, }, avatar: { width: 50, height: 50, borderRadius: 25, }, col: { marginLeft: 10, }, name: { fontSize: 16, color: '# 2e2e2e', }, email: { marginTop: 10, fontSize: 13, color: '# b0b0b0', }, });
Then we went back to App.js. Let’s replace the contents of the file with the following code:
import React, {Component} from 'react'; import {SafeAreaView} from 'react-native'; import {NavigationContainer} from '@ react-navigation / native'; import {RootNavigator} from './navigators/RootNavigator'; export class App extends Component { render = () => { return ( <SafeAreaView> <NavigationContainer> <RootNavigator /> </NavigationContainer> </SafeAreaView> ); }; } const styles = StyleSheet.create ({ container: { flex: 1, }, });
We use our created router so that navigation would work for us. The SafeAreaView component is also used here, it is needed so that the content in iOS is drawn inside the “safe” borders of the screen.
Build and launch
Let’s try to build and run what we have. If you have macOS, you can use Xcode. To do this, when you open a project, you need to specify the ios / RandomGuysApp.xcworkspace file inside the project. If it’s Android, then you need to install the JDK and the necessary SDKs. The easiest way is to open the Android folder in Android Studio and the IDE will install the required software. And then write react-native run-android in the root folder of the npx project.
When the project starts, on the screen some results will appear. This is the screen called list that we registered in the navigator. But it is still empty there, since we have nothing to draw yet. So let’s go back to PersonListScreen.js and add code to getMoreData () in order to get the data.
To debug the application, you can use react-native-debugger, or simply a browser. The debugger is enabled by the key combination ctrl (cmd) + M for Android and cmd + D for iOS. You can also enable live reload there.
On top of import from react-native, add the import of the StyleSheet module:
import {FlatList, View, StyleSheet} from 'react-native';
Let’s also create a file src / screens / PersonInfoScreen.js and add the following code there:
import React, {Component} from 'react'; import {StyleSheet, View, Image, Text} from 'react-native'; export class PersonInfoScreen extends Component { renderRow = (cells) => { return cells.map ((cell) => ( <View style={styles.cell} key = {cell.title}> <Text style={styles.cellTitle}> {cell.title} </Text> <Text style={styles.cellValue}> {cell.value} </Text> </View> )); }; render = () => { const {person} = this.props.route.params; return ( <View style={styles.container}> <Image source = {{uri: person.picture.large}} style={styles.avatar} resizeMode = {'contain'} /> {this.renderRow ([ {title: 'login', value: person.login.username}, {title: 'name', value: person.name.first}, {title: 'surname', value: person.name.last}, {title: 'email', value: person.email}, {title: 'phone', value: person.cell}, ])} </View> ); }; } const styles = StyleSheet.create ({ container: { flex: 1, alignItems: 'center', }, avatar: { width: 100, height: 100, borderRadius: 50, marginTop: 20, }, cell: { marginTop: 15, justifyContent: 'center', alignItems: 'center', }, cellTitle: { fontSize: 13, color: '# b0b0b0', }, cellValue: { marginTop: 10, fontSize: 16, color: '# 2e2e2e', }, });
So, that’s it! The application is almost ready to use.
Well, where can you get more knowledge about React Native? Actually, there are many resources to explore RN, here’s a short list:
- Official documentation, here you can find descriptions of all APIs and built-in library components.
- One of the best courses for learning React Native. It will help you quickly understand it, as well as teach you an understanding of the RN ecosystem and React in general.
- There is a large list of cool libraries for different occasions and articles on the topic.
Conclusion
To sum everything up, React Native is a powerful platform used by businesses of all sizes to build mobile apps and websites. It is a fast, efficient and relatively easy way to create JavaScript applications. Its main advantage is that it allows you to quickly create an application for several platforms at the same time. React Native is widely used by big brands, so don’t worry about it going anywhere in the next couple of years.
I hope that this article was a helpful guide for you in describing the process to build a React Native application. Or maybe you can look for a professional React Native development company.